Example 3: Urban climate adaption
[1]:
from pyclmuapp import usp_clmu
import matplotlib.pyplot as plt
Here we use the RUN_TYPE= "branch"
to avoid the repeated spinup phases.
The case (usp_spinup
) is run for 10 years to spinup the model.
How to get usp_spinup
?
usp_spinup = usp.run(
case_name = "usp_spinup",
SURF="surfdata.nc",
FORCING="forcing.nc",
RUN_STARTDATE = "2002-01-01",
STOP_OPTION = "nyears",
STOP_N = "10",
RUN_TYPE= "coldstart",
)
usp_spinup
[2]:
%%time
# initialize
usp = usp_clmu()
usp_or = usp.run(
case_name = "example3",
SURF="surfdata.nc",
FORCING="forcing.nc",
RUN_STARTDATE = "2012-01-01",
STOP_OPTION = "nyears",
STOP_N = "2",
RUN_TYPE= "branch",
RUN_REFCASE= "usp_spinup", # the case name of the spinup run
RUN_REFDATE= "2012-01-01",
)
usp_or
Copying the file forcing.nc to the /Users/user/Documents/GitHub/pyclmuapp/docs/notebooks/usp/workdir/inputfolder/usp
CPU times: user 1.04 s, sys: 268 ms, total: 1.31 s
Wall time: 2min 37s
[2]:
['/Users/user/Documents/GitHub/pyclmuapp/docs/notebooks/usp/workdir/outputfolder/lnd/hist/example3_clm0_2024-11-23_15-31-18_clm.nc']
2 explore the urban adaptation to urban climate
workflow:
case2: modify the urban roof albedo –> simulate the white/cooling roof for urban adaptation
case3: modify the forcing –> simulate global warming
case4: modify forcing and urban roof albedo –> white roof effect under global warming
how to change the surface data?
# change the surface data
usp_clmu.modify_surf(
action=action, # dict like result of usp_clmu.check_surf_data()
#or usp_clmu.surfdata_dict
mode="replace", # optional; the default is "replace"
usr_surfdata=None, # optional; the path to the new surfdata file,
#the default is "surfdata.nc" provided by pyclmuapp
surfata_name="surface_replaced.nc" # optional; output file name,
# the default is "surfdata.nc"
)
Args:
usr_surfdata (str): The path to the user-defined surface data file. The default is None.
action (dict): The dictionary of the revised surface data for the urban surface parameters. The default is None, which means no action.
mode (str): The mode for the revision. The default is “replace”.
surfata_name (str): The name of the revised surface data file. The default is “surfdata.nc”.
urban_type (int): The type of the urban surface. The default is 2. 0 is for TBD urban, 1 is for HD urban, and 2 is for MD urban.
[3]:
%%time
# we have run a simulation using the `usp` object,
# the forcing data path is stored in the `usp.usr_forcing_file` attribute
# the surface data path is stored in the `usp.surfdata` attribute
# we can modify the forcing data by calling the `usp.modify_forcing` method
# and run the simulation again using the `usp.run` method
# if there is no forcing data path provided or have not run a simulation using the `usp` object,
# check the forcing, by calling `usp.check_forcing(usr_forcing="forcing.nc")` method
# if there is no surface data path provided or have not run a simulation using the `usp` object,
# check the surface, by calling `usp.check_surf(usr_surf="sufdata.nc")` method
# modify the surface
usp.modify_surf(action={"ALB_ROOF_DIR":0.2}, surfata_name="surface_modfied.nc", mode="add")
usp.modify_surf(action={"ALB_ROOF_DIF":0.2}, surfata_name="surface_modfied.nc", mode="add")
usp_surf = usp.run(
case_name = "example3",
RUN_STARTDATE = "2012-01-01",
STOP_OPTION = "nyears",
STOP_N = "2",
RUN_TYPE= "branch",
RUN_REFCASE= "usp_spinup", # the case name of the spinup run
RUN_REFDATE= "2012-01-01",
)
# modify the forcing
usp.modify_forcing(action={"Tair": 1}, mode="add", forcing_name="forcing_replaced.nc")
usp_warming_surf = usp.run(
case_name = "example3",
RUN_STARTDATE = "2012-01-01",
STOP_OPTION = "nyears",
STOP_N = "2",
RUN_TYPE= "branch",
RUN_REFCASE= "usp_spinup", # the case name of the spinup run
RUN_REFDATE= "2012-01-01",
)
# recover the surface
usp.modify_surf(action={"ALB_ROOF_DIR":-0.2}, surfata_name="surface_modfied.nc", mode="add")
usp.modify_surf(action={"ALB_ROOF_DIF":-0.2}, surfata_name="surface_modfied.nc", mode="add")
usp_warming = usp.run(
case_name = "example3",
RUN_STARTDATE = "2012-01-01",
STOP_OPTION = "nyears",
STOP_N = "2",
RUN_TYPE= "branch",
RUN_REFCASE= "usp_spinup", # the case name of the spinup run
RUN_REFDATE= "2012-01-01",
)
# recover the forcing
usp.modify_forcing(action={"Tair": -1}, mode="add", forcing_name="forcing_replaced.nc")
CPU times: user 1.47 s, sys: 351 ms, total: 1.82 s
Wall time: 4min 38s
[4]:
print(usp_or)
print(usp_warming)
print(usp_surf)
print(usp_warming_surf)
['/Users/user/Documents/GitHub/pyclmuapp/docs/notebooks/usp/workdir/outputfolder/lnd/hist/example3_clm0_2024-11-23_15-31-18_clm.nc']
['/Users/user/Documents/GitHub/pyclmuapp/docs/notebooks/usp/workdir/outputfolder/lnd/hist/example3_clm0_2024-11-23_15-35-55_clm.nc']
['/Users/user/Documents/GitHub/pyclmuapp/docs/notebooks/usp/workdir/outputfolder/lnd/hist/example3_clm0_2024-11-23_15-32-50_clm.nc']
['/Users/user/Documents/GitHub/pyclmuapp/docs/notebooks/usp/workdir/outputfolder/lnd/hist/example3_clm0_2024-11-23_15-34-23_clm.nc']
use ``usp_clmu.nc_view()`` to read the output files
[5]:
ds_or_usp = usp.nc_view(usp_or[0])
ds_warming_usp = usp.nc_view(usp_warming[0])
ds_surf_usp = usp.nc_view(usp_surf[0])
ds_warming_surf_usp = usp.nc_view(usp_warming_surf[0])
3 Plotting
Note: ploting with time will need the nc-time-axis
package, which can be installed by pip install nc-time-axis
or conda install nc-time-axis
[6]:
fig = plt.figure(figsize=(6, 5))
ax = fig.add_subplot(111)
# plot the original
dd = (ds_surf_usp['TSA']-ds_or_usp['TSA']).isel(gridcell=0).groupby('time.hour')
mean = dd.mean('time')
var = dd.var('time')
mean.plot(ax=ax, label='Original', color='#3964DF')
ax.fill_between(mean['hour'].values, mean - var, mean + var, alpha=0.3, color='#3964DF')
# plot the warming
dd = (ds_warming_surf_usp['TSA']-ds_warming_usp['TSA']).isel(gridcell=0).groupby('time.hour')
mean = dd.mean('time')
var = dd.var('time')
mean.plot(ax=ax, label='Warming 1 K', color='#E02927')
ax.fill_between(mean['hour'].values, mean - var, mean + var, alpha=0.3, color='#E02927')
ax.set_xlabel('Local hour of day', fontsize=14)
ax.set_ylabel('Temperature difference [K]', fontsize=14)
ax.tick_params(axis='both', which='major', labelsize=12)
ax.set_title('')
ax.legend(frameon=False, fontsize=12)
plt.tight_layout()
plt.savefig('figs/example3_usp.pdf', dpi=300)
plt.show()
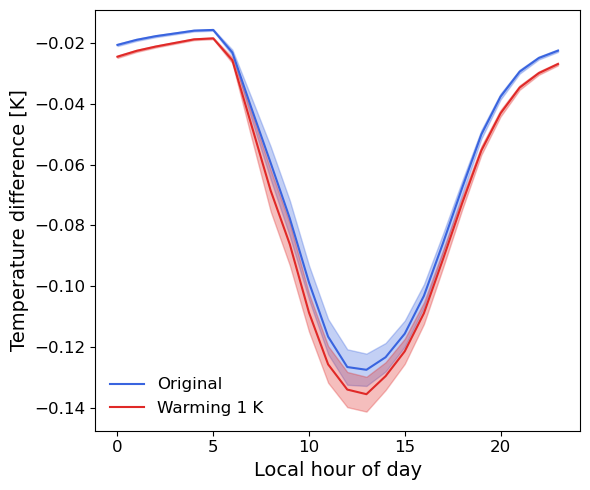
clean up the case files
[7]:
usp.case_clean(case_name="example3")
# when you using ups.run(crun_type='usp-exec') --> see warmup section
# the case files in ./workdir/ouptutfolder/your_case, ./workdir/logfolder/your_case,
# and ./workdir/inputfoler/usp will be removed.
# when you using ups.run(crun_type='run') or ups.run(), the default crun_type is 'run'
# only ./workdir/inputfoler/usp will be removed.